Added M0110A support contributed by skagon@github.
- README is written with markdown notation. - m0110.c can handles Arrow keys and Calc keys of M0110A. - EXTRAFLAGS and EXTRALDFLAGS are added in rules.mk to give flags on make command line.
This commit is contained in:
parent
0a4fa89548
commit
12f6e9ffa7
81
m0110.c
81
m0110.c
@ -34,6 +34,7 @@ CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
|
||||
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
|
||||
POSSIBILITY OF SUCH DAMAGE.
|
||||
*/
|
||||
/* M0110A Support was contributed by skagon@github */
|
||||
|
||||
#include <stdbool.h>
|
||||
#include <avr/io.h>
|
||||
@ -43,6 +44,8 @@ POSSIBILITY OF SUCH DAMAGE.
|
||||
#include "debug.h"
|
||||
|
||||
|
||||
static inline uint8_t inquiry(void);
|
||||
static inline uint8_t instant(void);
|
||||
static inline void clock_lo(void);
|
||||
static inline void clock_hi(void);
|
||||
static inline bool clock_in(void);
|
||||
@ -106,6 +109,23 @@ KEY EVENT:
|
||||
Moreover, the numpad keys =, /, * and + are preceded by shift-down 0x71 on press and shift-up 0xF1 on release.
|
||||
So, the data transferred by nupmad 5 is "79 2F" whereas for numpad + it's "71 79 0D".
|
||||
|
||||
ARROW KEYS:
|
||||
Arrow keys and Pad+,*,/,=(Calc keys) share same byte sequence and its preceding byte
|
||||
0x71 and 0xF1 means press and release event of SHIFT. These cause very confusing situation.
|
||||
It is difficult or impossible to tell Calc key from Arrow key with SHIFT in some cases.
|
||||
|
||||
Raw key events:
|
||||
press release
|
||||
---------------- ----------------
|
||||
Left: 0x79, 0x0D 0x79, 0x8D
|
||||
Right: 0x79, 0x05 0x79, 0x85
|
||||
Up: 0x79, 0x1B 0x79, 0x9B
|
||||
Down: 0x79, 0x11 0x79, 0x91
|
||||
Pad+: 0x71, 0x79, 0x0D 0xF1, 0x79, 0x8D
|
||||
Pad*: 0x71, 0x79, 0x05 0xF1, 0x79, 0x85
|
||||
Pad/: 0x71, 0x79, 0x1B 0xF1, 0x79, 0x9B
|
||||
Pad=: 0x71, 0x79, 0x11 0xF1, 0x79, 0x91
|
||||
|
||||
SCAN CODE:
|
||||
m0111_recv_key() function returns follwing scan codes instead of raw key events.
|
||||
Scan codes are 1 byte long and bit7 is set when key is released.
|
||||
@ -275,16 +295,63 @@ ERROR:
|
||||
|
||||
uint8_t m0110_recv_key(void)
|
||||
{
|
||||
uint8_t key;
|
||||
m0110_send(M0110_INQUIRY);
|
||||
key = m0110_recv();
|
||||
if (key == 0xFF || key == M0110_NULL)
|
||||
return M0110_NULL;
|
||||
else
|
||||
return M0110_RAW2SCAN(key);
|
||||
static uint8_t keybuf = 0x00;
|
||||
uint8_t key, key2, key3;
|
||||
|
||||
if (keybuf) {
|
||||
key = keybuf;
|
||||
keybuf = 0x00;
|
||||
return key;
|
||||
}
|
||||
key = instant(); // Use INSTANT for better response. Should be INQUIRY ?
|
||||
switch (key) {
|
||||
case M0110_KEYPAD:
|
||||
// Pad/Arrow keys
|
||||
return (M0110_RAW2SCAN(instant()) | M0110_KEYPAD_OFFSET);
|
||||
break;
|
||||
case M0110_SHIFT_MAKE:
|
||||
case M0110_SHIFT_BREAK:
|
||||
key2 = instant();
|
||||
if (key2 == M0110_KEYPAD) {
|
||||
key3 = instant();
|
||||
switch (key3) {
|
||||
case M0110_ARROW_UP:
|
||||
case M0110_ARROW_DOWN:
|
||||
case M0110_ARROW_LEFT:
|
||||
case M0110_ARROW_RIGHT:
|
||||
// Calc keys
|
||||
return (M0110_RAW2SCAN(key3) | M0110_CALC_OFFSET);
|
||||
default:
|
||||
// Shift + Pad/Arrow keys
|
||||
keybuf = M0110_RAW2SCAN(key3);
|
||||
return (M0110_RAW2SCAN(key) | M0110_KEYPAD_OFFSET);
|
||||
}
|
||||
} else {
|
||||
// Shift + other keys
|
||||
keybuf = M0110_RAW2SCAN(key2);
|
||||
return M0110_RAW2SCAN(key);
|
||||
}
|
||||
break;
|
||||
default:
|
||||
// other keys
|
||||
return M0110_RAW2SCAN(key);
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
static inline uint8_t inquiry(void)
|
||||
{
|
||||
m0110_send(M0110_INQUIRY);
|
||||
return m0110_recv();
|
||||
}
|
||||
|
||||
static inline uint8_t instant(void)
|
||||
{
|
||||
m0110_send(M0110_INSTANT);
|
||||
return m0110_recv();
|
||||
}
|
||||
|
||||
static inline void clock_lo()
|
||||
{
|
||||
M0110_CLOCK_PORT &= ~(1<<M0110_CLOCK_BIT);
|
||||
|
38
m0110.h
38
m0110.h
@ -54,23 +54,39 @@ POSSIBILITY OF SUCH DAMAGE.
|
||||
# error "M0110 data port setting is required in config.h"
|
||||
#endif
|
||||
|
||||
#define M0110_INQUIRY 0x10
|
||||
#define M0110_INSTANT 0x14
|
||||
#define M0110_MODEL 0x16
|
||||
#define M0110_TEST 0x36
|
||||
/* Commands */
|
||||
#define M0110_INQUIRY 0x10
|
||||
#define M0110_INSTANT 0x14
|
||||
#define M0110_MODEL 0x16
|
||||
#define M0110_TEST 0x36
|
||||
|
||||
#define M0110_PAD 0x79
|
||||
#define M0110_NULL 0x7B
|
||||
#define M0110_TEST_ACK 0x7D
|
||||
#define M0110_TEST_NAK 0x77
|
||||
/* Response(raw byte from M0110) */
|
||||
#define M0110_NULL 0x7B
|
||||
#define M0110_KEYPAD 0x79
|
||||
#define M0110_TEST_ACK 0x7D
|
||||
#define M0110_TEST_NAK 0x77
|
||||
#define M0110_SHIFT_MAKE 0x71
|
||||
#define M0110_SHIFT_BREAK 0xF1
|
||||
#define M0110_ARROW_UP 0x1B
|
||||
#define M0110_ARROW_DOWN 0x11
|
||||
#define M0110_ARROW_LEFT 0x0D
|
||||
#define M0110_ARROW_RIGHT 0x05
|
||||
|
||||
/* This inidcates no response. */
|
||||
#define M0110_ERROR 0xFF
|
||||
|
||||
/* scan code offset for keypad and arrow keys */
|
||||
#define M0110_KEYPAD_OFFSET 0x40
|
||||
#define M0110_ARROW_OFFSET 0x60
|
||||
#define M0110_CALC_OFFSET 0x60
|
||||
|
||||
/* convert key event raw response into scan code */
|
||||
#define M0110_RAW2SCAN(key) ((key&(1<<7)) | ((key&0x7F)>>1))
|
||||
#define M0110_RAW2SCAN(key) ( \
|
||||
(key == M0110_NULL) ? M0110_NULL : ( \
|
||||
(key == M0110_ERROR) ? M0110_ERROR : ( \
|
||||
((key&0x80) | ((key&0x7F)>>1)) \
|
||||
) \
|
||||
) \
|
||||
)
|
||||
|
||||
|
||||
extern uint8_t m0110_error;
|
||||
@ -80,5 +96,7 @@ void m0110_init(void);
|
||||
uint8_t m0110_send(uint8_t data);
|
||||
uint8_t m0110_recv(void);
|
||||
uint8_t m0110_recv_key(void);
|
||||
uint8_t m0110_inquiry(void);
|
||||
uint8_t m0110_instant(void);
|
||||
|
||||
#endif
|
||||
|
@ -1,55 +0,0 @@
|
||||
M0110 to USB keyboard converter
|
||||
===============================
|
||||
This firmware converts the protocol of Apple Macintosh keyboard M0110 into USB.
|
||||
|
||||
|
||||
Connection
|
||||
----------
|
||||
You need 4P4C plug and cable to connect Teensy into M0110.
|
||||
Teensy port F0 is assigned for CLOCK line and F1 for DATA by default, you can change pin configuration with editing config.h..
|
||||
|
||||
Plug:
|
||||
http://en.wikipedia.org/wiki/Modular_connector#4P4C
|
||||
|
||||
Pinout:
|
||||
http://www.kbdbabel.org/conn/kbd_connector_macplus.png
|
||||
1(Black): GND
|
||||
2(Red): CLOCK
|
||||
3(Green): DATA
|
||||
4(Yellow): +5V
|
||||
|
||||
|
||||
|
||||
Build Frimware
|
||||
--------------
|
||||
Optionally edit Makefile and config.h for build options, pin configuration or MCU.
|
||||
|
||||
$ cd m0110_usb
|
||||
$ make
|
||||
and program your Teensy with loader.
|
||||
|
||||
|
||||
|
||||
Keymap
|
||||
------
|
||||
You can change a keymap by editing code of keymap.c like following.
|
||||
How to define the keymap is probably obvious. You can find key symbols in usb_keycodes.h.
|
||||
|
||||
This is a default keymap for M0110.
|
||||
,---------------------------------------------------------.
|
||||
| `| 1| 2| 3| 4| 5| 6| 7| 8| 9| 0| -| =|Bacpa|
|
||||
|---------------------------------------------------------|
|
||||
|Tab | Q| W| E| R| T| Y| U| I| O| P| [| ]| \|
|
||||
|---------------------------------------------------------|
|
||||
|CapsLo| A| S| D| F| G| H| J| K| L| ;| '|Return|
|
||||
|---------------------------------------------------------|
|
||||
|Shift | Z| X| C| V| B| N| M| ,| ,| /|Shift |
|
||||
`---------------------------------------------------------'
|
||||
|Opt|Alt | Space |Alt |Opt|
|
||||
`-----------------------------------------------'
|
||||
|
||||
|
||||
Notes
|
||||
-----
|
||||
|
||||
EOF
|
141
m0110_usb/README.md
Normal file
141
m0110_usb/README.md
Normal file
@ -0,0 +1,141 @@
|
||||
M0110/M0110A to USB keyboard converter
|
||||
======================================
|
||||
This firmware converts the protocol of Apple Macintosh keyboard M0110/M0110A into USB.
|
||||
Target board of this project is [PJRC Teensy](http://www.pjrc.com/teensy/), though,
|
||||
you can use other board with USB AVR like `ATmega32U4` and `AT90USB`.
|
||||
|
||||

|
||||
|
||||
M0110A support was contributed by [skagon@github](https://github.com/skagon).
|
||||
|
||||
|
||||
|
||||
Connection
|
||||
----------
|
||||
You need 4P4C plug and cable to connect Teensy or other AVR dev board into the keyboard.
|
||||
Teensy port `PF0` is assigned for `CLOCK` line and `PF1` for `DATA` by default,
|
||||
you can change pin configuration with editing *config.h*.
|
||||
|
||||
You can find 4P4C plugs on telephone handset cable. Note that it is *crossover* connection
|
||||
while Macintosh keyboard cable is *straight*.
|
||||
|
||||
[](http://i.imgur.com/vJoVO.jpg)
|
||||
|
||||
In this pic:
|
||||
|
||||
1. `GND`(Black)
|
||||
2. `CLOCK`(Red)
|
||||
3. `DATA`(Green)
|
||||
4. `+5V`(Yellow)
|
||||
|
||||
Not that wire colors may vary in your cable.
|
||||
|
||||
|
||||
### Pinout
|
||||
- <http://pinouts.ru/Inputs/MacKeyboard_pinout.shtml>
|
||||
- <http://en.wikipedia.org/wiki/Modular_connector#4P4C>
|
||||
|
||||
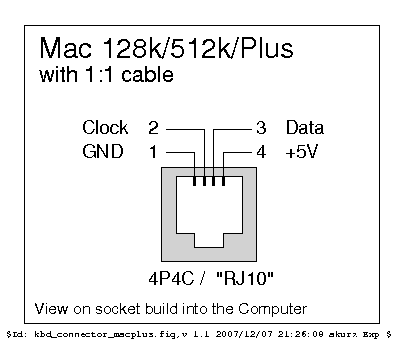
|
||||
|
||||
|
||||
### Pull-up Registor
|
||||
You may need pull-up registors on signal lines(`CLOCK`, `DATA`) in particular
|
||||
when you have long or coiled cable. 1k-10k Ohm will be OK for this purpose.
|
||||
In some cases MCU can't read signal from keyboard correctly without pull-up resistors.
|
||||
|
||||
|
||||
|
||||
Building Frimware
|
||||
-----------------
|
||||
To compile firmware you need AVR GCC. You can use [WinAVR](http://winavr.sourceforge.net/) on Windows.
|
||||
You can edit *Makefile* and *config.h* to change compile options and pin configuration.
|
||||
|
||||
$ git clone ... (or download source)
|
||||
$ cd m0110_usb
|
||||
$ make
|
||||
|
||||
and program your Teensy with [PJRC Teensy loader](http://www.pjrc.com/teensy/loader.html).
|
||||
|
||||
|
||||
|
||||
Keymap
|
||||
------
|
||||
You can change keymaps by editing *keymap.c*.
|
||||
|
||||
### M0110
|
||||
#### *Default*
|
||||
,---------------------------------------------------------.
|
||||
| `| 1| 2| 3| 4| 5| 6| 7| 8| 9| 0| -| =|Backs|
|
||||
|---------------------------------------------------------|
|
||||
|Tab | Q| W| E| R| T| Y| U| I| O| P| [| ]| \|
|
||||
|---------------------------------------------------------|
|
||||
|Fn0 | A| S| D| F| G| H| J| K| L| ;| '|Return|
|
||||
|---------------------------------------------------------|
|
||||
|Shift | Z| X| C| V| B| N| M| ,| ,| /|Shift |
|
||||
`---------------------------------------------------------'
|
||||
|Ctr|Alt | Space |Gui |Ctr|
|
||||
`-----------------------------------------------'
|
||||
You can register Esc by hitting(press&release) Fn0 quickly.
|
||||
|
||||
#### *HHKB/WASD cursor Layer(Fn0)*
|
||||
,---------------------------------------------------------.
|
||||
|Esc| F1| F2| F3| F4| F5| F6| F7| F8| F9|F10|F11|F12|Delet|
|
||||
|---------------------------------------------------------|
|
||||
|Caps |Hom| Up|PgU| | | | |Psc|Slk|Pau|Up |Ins| \|
|
||||
|---------------------------------------------------------|
|
||||
|Fn0 |Lef|Dow|Rig| | | | |Hom|PgU|Lef|Rig|Return|
|
||||
|---------------------------------------------------------|
|
||||
|Shift |End| |PgD| |VoD|VoU|Mut|End|PgD|Dow|Shift |
|
||||
`---------------------------------------------------------'
|
||||
|Ctr|Alt | Space |Gui |Ctr|
|
||||
`-----------------------------------------------'
|
||||
|
||||
### M0110A
|
||||
#### *Default*
|
||||
,---------------------------------------------------------. ,---------------.
|
||||
| `| 1| 2| 3| 4| 5| 6| 7| 8| 9| 0| -| =|Backs| |Gui| =| /| *|
|
||||
|---------------------------------------------------------| |---------------|
|
||||
|Tab | Q| W| E| R| T| Y| U| I| O| P| [| ]| | | 7| 8| 9| -|
|
||||
|-----------------------------------------------------' | |---------------|
|
||||
|Fn0 | A| S| D| F| G| H| J| K| L| ;| '|Return| | 4| 5| 6| +|
|
||||
|---------------------------------------------------------| |---------------|
|
||||
|Shift | Z| X| C| V| B| N| M| ,| ,| /|Shft|Up | | 1| 2| 3| |
|
||||
|---------------------------------------------------------| |-----------|Ent|
|
||||
|Ctrl |Alt | Space | \|Lft|Rgt|Dn | | 0| .| |
|
||||
`---------------------------------------------------------' `---------------'
|
||||
#### *HHKB/WASD cursor Layer(Fn0)*
|
||||
,---------------------------------------------------------. ,---------------.
|
||||
|Esc| F1| F2| F3| F4| F5| F6| F7| F8| F9|F10|F11|F12|Delet| |Nlk| =| /| *|
|
||||
|---------------------------------------------------------| |---------------|
|
||||
|Caps |Hom| Up|PgU| | | | |Psc|Slk|Pau|Up |Ins| | | 7| 8| 9| -|
|
||||
|-----------------------------------------------------' | |---------------|
|
||||
|Fn0 |Lef|Dow|Rig| | | | |Hom|PgU|Lef|Rig|Return| | 4| 5| 6| +|
|
||||
|---------------------------------------------------------| |---------------|
|
||||
|Shift |End| |PgD| |VoD|VoU|Mut|End|PgD|Dow|Shif|Up | | 1| 2| 3| |
|
||||
|---------------------------------------------------------| |-----------|Ent|
|
||||
|Ctrl |Alt | Space | \|Lft|Rgt|Dn | | 0| .| |
|
||||
`---------------------------------------------------------' `---------------'
|
||||
|
||||
|
||||
|
||||
Debug
|
||||
-----
|
||||
You can use [PJRC HID listen](http://www.pjrc.com/teensy/hid_listen.html) to see debug output.
|
||||
|
||||
The converter has some functions for debug, press `Alt+Gui+H` simultaneously to get help.
|
||||
These function is totally undocumented, tentative, inconsistent and buggy.
|
||||
|
||||
|
||||
|
||||
Arrow Keys
|
||||
----------
|
||||
Dedicated arrow keys of the M0110A are transmitting the same scancodes as the keypad but also,
|
||||
its [=], [/], [*] and [+] keys (hereafter referred to as "calc" keys) are not assigned new
|
||||
scancodes but, instead, transmit a sequence of scancodes which emulates the [Shift] key press,
|
||||
followed by the same scancode sequence of the arrow keys!
|
||||
The problem with that approach is that, while in most cases it's easy to distinguish between
|
||||
a user-generated [Shift] key event (press or release) followed by an arrow or a calc key and
|
||||
a simulated [Shift] key event generated upon a calc key event, when the user is typing fairly
|
||||
fast, it is possible that the two events become indistinguishable, and produce undesired results
|
||||
-- nothing major, though, just one or two stray characters or cursor movements; it will NOT
|
||||
format your drives, kill your cat or make your wife run away with the pizza boy.
|
@ -30,7 +30,7 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
|
||||
|
||||
/* matrix size */
|
||||
#define MATRIX_ROWS 8
|
||||
#define MATRIX_ROWS 14
|
||||
#define MATRIX_COLS 8
|
||||
|
||||
/* Locking Caps Lock support */
|
||||
@ -38,8 +38,7 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
|
||||
/* key combination for command */
|
||||
#define IS_COMMAND() ( \
|
||||
keyboard_report->mods == (MOD_BIT(KB_LSHIFT) | MOD_BIT(KB_LCTRL) | MOD_BIT(KB_LALT) | MOD_BIT(KB_LGUI)) || \
|
||||
keyboard_report->mods == (MOD_BIT(KB_LSHIFT) | MOD_BIT(KB_RSHIFT)) \
|
||||
keyboard_report->mods == (MOD_BIT(KB_LALT) | MOD_BIT(KB_LGUI)) \
|
||||
)
|
||||
|
||||
|
||||
|
@ -14,10 +14,8 @@ GNU General Public License for more details.
|
||||
You should have received a copy of the GNU General Public License
|
||||
along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
*/
|
||||
/* M0110A Support was contributed by skagon@github */
|
||||
|
||||
/*
|
||||
* Keymap for ADB keyboard
|
||||
*/
|
||||
#include <stdint.h>
|
||||
#include <stdbool.h>
|
||||
#include <avr/pgmspace.h>
|
||||
@ -31,16 +29,12 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
|
||||
#define KEYCODE(layer, row, col) (pgm_read_byte(&keymaps[(layer)][(row)][(col)]))
|
||||
|
||||
// Convert physical keyboard layout to matrix array.
|
||||
// This is a macro to define keymap easily in keyboard layout form.
|
||||
// TODO: layout for M0110A
|
||||
/* M0110 */
|
||||
#define KEYMAP( \
|
||||
K32,K12,K13,K14,K15,K17,K16,K1A,K1C,K19,K1D,K1B,K18,K33, \
|
||||
K30,K0C,K0D,K0E,K0F,K11,K10,K20,K22,K1F,K23,K21,K1E,K2A, \
|
||||
K39,K00,K01,K02,K03,K05,K04,K26,K28,K25,K29,K27, K24, \
|
||||
K38,K06,K07,K08,K09,K0B,K2D,K2E,K2B,K2F,K2C, \
|
||||
K3A,K37, K31, K34 \
|
||||
K32,K12,K13,K14,K15,K17,K16,K1A,K1C,K19,K1D,K1B,K18,K33, K47,K68,K6D,K62, \
|
||||
K30,K0C,K0D,K0E,K0F,K11,K10,K20,K22,K1F,K23,K21,K1E, K59,K5B,K5C,K4E, \
|
||||
K39,K00,K01,K02,K03,K05,K04,K26,K28,K25,K29,K27, K24, K56,K57,K58,K66, \
|
||||
K38,K06,K07,K08,K09,K0B,K2D,K2E,K2B,K2F,K2C, K4D, K53,K54,K55,K4C, \
|
||||
K3A,K37, K31, K34,K2A,K46,K42,K48, K52, K41 \
|
||||
) { \
|
||||
{ KB_##K00, KB_##K01, KB_##K02, KB_##K03, KB_##K04, KB_##K05, KB_##K06, KB_##K07 }, \
|
||||
{ KB_##K08, KB_##K09, KB_NO, KB_##K0B, KB_##K0C, KB_##K0D, KB_##K0E, KB_##K0F }, \
|
||||
@ -49,7 +43,13 @@ along with this program. If not, see <http://www.gnu.org/licenses/>.
|
||||
{ KB_##K20, KB_##K21, KB_##K22, KB_##K23, KB_##K24, KB_##K25, KB_##K26, KB_##K27 }, \
|
||||
{ KB_##K28, KB_##K29, KB_##K2A, KB_##K2B, KB_##K2C, KB_##K2D, KB_##K2E, KB_##K2F }, \
|
||||
{ KB_##K30, KB_##K31, KB_##K32, KB_##K33, KB_##K34, KB_NO, KB_NO, KB_##K37 }, \
|
||||
{ KB_##K38, KB_##K39, KB_##K3A, KB_NO, KB_NO, KB_NO, KB_NO, KB_NO } \
|
||||
{ KB_##K38, KB_##K39, KB_##K3A, KB_NO, KB_NO, KB_NO, KB_NO, KB_NO }, \
|
||||
{ KB_NO, KB_##K41, KB_##K42, KB_NO, KB_NO, KB_NO, KB_##K46, KB_##K47 }, \
|
||||
{ KB_##K48, KB_NO, KB_NO, KB_NO, KB_##K4C, KB_##K4D, KB_##K4E, KB_NO }, \
|
||||
{ KB_NO, KB_NO, KB_##K52, KB_##K53, KB_##K54, KB_##K55, KB_##K56, KB_##K57 }, \
|
||||
{ KB_##K58, KB_##K59, KB_NO, KB_##K5B, KB_##K5C, KB_NO, KB_NO, KB_NO }, \
|
||||
{ KB_NO, KB_NO, KB_##K62, KB_NO, KB_NO, KB_NO, KB_##K66, KB_NO }, \
|
||||
{ KB_##K68, KB_NO, KB_NO, KB_NO, KB_NO, KB_##K6D, KB_NO, KB_NO }, \
|
||||
}
|
||||
|
||||
|
||||
@ -68,9 +68,14 @@ static const uint8_t PROGMEM fn_layer[] = {
|
||||
// Assign Fn key(0-7) to a keycode sent when release Fn key without use of the layer.
|
||||
// See layer.c for details.
|
||||
static const uint8_t PROGMEM fn_keycode[] = {
|
||||
KB_SCOLON, // Fn0
|
||||
KB_SLASH, // Fn1
|
||||
KB_ESC, // Fn0
|
||||
#ifdef HASU
|
||||
KB_SCOLON, // Fn1
|
||||
KB_SLASH, // Fn2
|
||||
#else
|
||||
KB_NO, // Fn1
|
||||
KB_NO, // Fn2
|
||||
#endif
|
||||
KB_NO, // Fn3
|
||||
KB_NO, // Fn4
|
||||
KB_NO, // Fn5
|
||||
@ -79,52 +84,97 @@ static const uint8_t PROGMEM fn_keycode[] = {
|
||||
};
|
||||
|
||||
static const uint8_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
|
||||
// LShift and RShift are logically same one button.
|
||||
// LOption and ROption are logically same one button.
|
||||
/* Default Layer: plain keymap
|
||||
* ,---------------------------------------------------------.
|
||||
* | `| 1| 2| 3| 4| 5| 6| 7| 8| 9| 0| -| =|Bacpa|
|
||||
* |---------------------------------------------------------|
|
||||
* |Tab | Q| W| E| R| T| Y| U| I| O| P| [| ]| \|
|
||||
* |---------------------------------------------------------|
|
||||
* |Contro| A| S| D| F| G| H| J| K| L|Fn0| '|Return|
|
||||
* |---------------------------------------------------------|
|
||||
* |Shift | Z| X| C| V| B| N| M| ,| ,|Fn1| Shift|
|
||||
* `---------------------------------------------------------'
|
||||
* |Fn2|Alt | Space |Gui |Fn2|
|
||||
* `-----------------------------------------------'
|
||||
/*
|
||||
* The keymap works with both M0110 and M0110A keyboards. As you can see, the M0110A is a superset
|
||||
* of the M0110 keyboard, with only one exception: the right Alt key(Enter in M0110) does not exist
|
||||
* on the M0110A, but since it generates a unique scan code which is not used for some other key in
|
||||
* the M0110A, they are totally interchangeable. In fact, the M0110A is functionally (almost)
|
||||
* identical to the combination of the M0110 along with the M0120 keypad. The only difference
|
||||
* (which is causing some problems as you will read below) is that the M0110+M0120 don't have
|
||||
* dedicated arrow keys, while the M0110A does. However, the M0120 did have arrow keys, which
|
||||
* doubled as the [comma], [/], [*] and [+] keys, when used with the [Shift] key. The M0110A has
|
||||
* substituted the [comma] key with the [=] key, however its scancode is the same.
|
||||
*
|
||||
* Default:
|
||||
* ,---------------------------------------------------------. ,---------------.
|
||||
* | `| 1| 2| 3| 4| 5| 6| 7| 8| 9| 0| -| =|Backs| |Gui| =| /| *|
|
||||
* |---------------------------------------------------------| |---------------|
|
||||
* |Tab | Q| W| E| R| T| Y| U| I| O| P| [| ]| | | 7| 8| 9| -|
|
||||
* |-----------------------------------------------------' | |---------------|
|
||||
* |Fn0 | A| S| D| F| G| H| J| K| L| ;| '|Return| | 4| 5| 6| +|
|
||||
* |---------------------------------------------------------| |---------------|
|
||||
* |Shift | Z| X| C| V| B| N| M| ,| ,| /|Shft|Up | | 1| 2| 3| |
|
||||
* |---------------------------------------------------------| |-----------|Ent|
|
||||
* |Ctrl |Alt | Space |Gui| \|Lft|Rgt|Dn | | 0| .| |
|
||||
* `---------------------------------------------------------' `---------------'
|
||||
* You can register Esc by hitting(press&release) Fn0 quickly.
|
||||
*
|
||||
* HHKB/WASD cursor Layer(Fn0):
|
||||
* ,---------------------------------------------------------. ,---------------.
|
||||
* |Esc| F1| F2| F3| F4| F5| F6| F7| F8| F9|F10|F11|F12|Delet| |Nlk| =| /| *|
|
||||
* |---------------------------------------------------------| |---------------|
|
||||
* |Caps |Hom| Up|PgU| | | | |Psc|Slk|Pau|Up |Ins| | | 7| 8| 9| -|
|
||||
* |-----------------------------------------------------' | |---------------|
|
||||
* |Fn0 |Lef|Dow|Rig| | | | |Hom|PgU|Lef|Rig|Return| | 4| 5| 6| +|
|
||||
* |---------------------------------------------------------| |---------------|
|
||||
* |Shift |End| |PgD| |VoD|VoU|Mut|End|PgD|Dow|Shif|Up | | 1| 2| 3| |
|
||||
* |---------------------------------------------------------| |-----------|Ent|
|
||||
* |Ctrl |Alt | Space |Gui | \|Lft|Rgt|Dn | | 0| .| |
|
||||
* `---------------------------------------------------------' `---------------'
|
||||
*
|
||||
* NOTE: Key between Space and \ in above diagram is M0110 Enter(assigned to Gui).
|
||||
* NOTE: LShift and RShift are logically same key. (M0110, M0110A)
|
||||
* NOTE: LOption and ROption are logically same key. (M0110)
|
||||
*/
|
||||
#ifdef HASU
|
||||
KEYMAP(
|
||||
GRV, 1, 2, 3, 4, 5, 6, 7, 8, 9, 0, MINS,EQL, BSPC,
|
||||
TAB, Q, W, E, R, T, Y, U, I, O, P, LBRC,RBRC,BSLS,
|
||||
LCTL,A, S, D, F, G, H, J, K, L, FN0, QUOT, ENT,
|
||||
LSFT,Z, X, C, V, B, N, M, COMM,DOT, FN1,
|
||||
FN2, LALT, SPC, LGUI
|
||||
),
|
||||
// vi mousekeys
|
||||
KEYMAP(
|
||||
ESC, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL,
|
||||
CAPS,NO, NO, NO, NO, NO, WH_L,WH_D,WH_U,WH_R,NO, NO, NO, NO,
|
||||
LCTL,VOLD,VOLU,MUTE,NO, NO, MS_L,MS_D,MS_U,MS_R,FN0, NO, ENT,
|
||||
LSFT,NO, NO, NO, NO, BTN3,BTN2,BTN1,NO, NO, NO,
|
||||
NO, LALT, BTN1, LGUI
|
||||
),
|
||||
// vi cusorkeys
|
||||
KEYMAP(
|
||||
ESC, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL,
|
||||
CAPS,NO, NO, NO, NO, NO, HOME,PGDN,PGUP,END, NO, NO, NO, NO,
|
||||
LCTL,NO, NO, NO, NO, NO, LEFT,DOWN,UP, RGHT,NO, NO, ENT,
|
||||
LSFT,NO, NO, NO, NO, NO, HOME,PGDN,PGUP,END, FN1,
|
||||
NO, LALT, SPC, LGUI
|
||||
ESC, 1, 2, 3, 4, 5, 6, 7, 8, 9, 0, MINS,EQL, BSPC, ESC, PEQL,PSLS,PAST,
|
||||
TAB, Q, W, E, R, T, Y, U, I, O, P, LBRC,RBRC, P7, P8, P9, PMNS,
|
||||
LCTL,A, S, D, F, G, H, J, K, L, FN1, QUOT, ENT, P4, P5, P6, PPLS,
|
||||
LSFT,Z, X, C, V, B, N, M, COMM,DOT, FN2, UP, P1, P2, P3, PENT,
|
||||
FN0, LALT, SPC, LGUI,BSLS,LEFT,DOWN,RGHT, P0, PDOT
|
||||
),
|
||||
// HHKB & WASD
|
||||
KEYMAP(
|
||||
ESC, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL,
|
||||
CAPS,HOME,UP, PGUP,NO, NO, NO, NO, PSCR,SLCK,BRK, UP, NO, NO,
|
||||
LCTL,LEFT,DOWN,RGHT,NO, NO, NO, NO, HOME,PGUP,LEFT,RGHT, ENT,
|
||||
LSFT,END, NO, PGDN,NO, VOLD,VOLU,MUTE,END, PGDN,DOWN,
|
||||
FN2, LALT, SPC, LGUI
|
||||
GRV, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL, NLCK,PEQL,PSLS,PAST,
|
||||
CAPS,HOME,UP, PGUP,NO, NO, NO, NO, PSCR,SLCK,BRK, UP, INS, P7, P8, P9, PMNS,
|
||||
LCTL,LEFT,DOWN,RGHT,NO, NO, NO, NO, HOME,PGUP,LEFT,RGHT, ENT, P4, P5, P6, PPLS,
|
||||
LSFT,END, NO, PGDN,NO, VOLD,VOLU,MUTE,END, PGDN,DOWN, UP, P1, P2, P3, PENT,
|
||||
FN0, LALT, SPC, LGUI,BSLS,LEFT,DOWN,RGHT, P0, PDOT
|
||||
),
|
||||
// vi mousekeys
|
||||
KEYMAP(
|
||||
GRV, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL, NLCK,PEQL,PSLS,PAST,
|
||||
CAPS,NO, NO, NO, NO, NO, WH_L,WH_D,WH_U,WH_R,NO, NO, NO, P7, P8, P9, PMNS,
|
||||
NO, VOLD,VOLU,MUTE,NO, NO, MS_L,MS_D,MS_U,MS_R,FN1, NO, ENT, P4, P5, P6, PPLS,
|
||||
LSFT,NO, NO, NO, NO, BTN3,BTN2,BTN1,NO, NO, NO, UP, P1, P2, P3, PENT,
|
||||
LCTL,LALT, BTN1, LGUI,BSLS,LEFT,DOWN,RGHT, P0, PDOT
|
||||
),
|
||||
// vi cusorkeys
|
||||
KEYMAP(
|
||||
GRV, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL, NLCK,PEQL,PSLS,PAST,
|
||||
CAPS,NO, NO, NO, NO, NO, HOME,PGDN,PGUP,END, NO, NO, NO, P7, P8, P9, PMNS,
|
||||
NO, NO, NO, NO, NO, NO, LEFT,DOWN,UP, RGHT,NO, NO, ENT, P4, P5, P6, PPLS,
|
||||
LSFT,NO, NO, NO, NO, NO, HOME,PGDN,PGUP,END, FN2, UP, P1, P2, P3, PENT,
|
||||
LCTL,LALT, SPC, LGUI,BSLS,LEFT,DOWN,RGHT, P0, PDOT
|
||||
),
|
||||
#else
|
||||
KEYMAP(
|
||||
GRV, 1, 2, 3, 4, 5, 6, 7, 8, 9, 0, MINS,EQL, BSPC, LGUI,PEQL,PSLS,PAST,
|
||||
TAB, Q, W, E, R, T, Y, U, I, O, P, LBRC,RBRC, P7, P8, P9, PMNS,
|
||||
FN0, A, S, D, F, G, H, J, K, L, SCLN,QUOT, ENT, P4, P5, P6, PPLS,
|
||||
LSFT,Z, X, C, V, B, N, M, COMM,DOT, SLSH, UP, P1, P2, P3, PENT,
|
||||
LCTL,LALT, SPC, LGUI,BSLS,LEFT,DOWN,RGHT, P0, PDOT
|
||||
),
|
||||
// HHKB & WASD
|
||||
KEYMAP(
|
||||
ESC, F1, F2, F3, F4, F5, F6, F7, F8, F9, F10, F11, F12, DEL, NLCK,PEQL,PSLS,PAST,
|
||||
CAPS,HOME,UP, PGUP,NO, NO, NO, NO, PSCR,SLCK,BRK, UP, INS, P7, P8, P9, PMNS,
|
||||
FN0, LEFT,DOWN,RGHT,NO, NO, NO, NO, HOME,PGUP,LEFT,RGHT, ENT, P4, P5, P6, PPLS,
|
||||
LSFT,END, NO, PGDN,NO, VOLD,VOLU,MUTE,END, PGDN,DOWN, UP, P1, P2, P3, PENT,
|
||||
LCTL,LALT, SPC, LGUI,BSLS,LEFT,DOWN,RGHT, P0, PDOT
|
||||
),
|
||||
#endif
|
||||
};
|
||||
|
||||
|
||||
|
@ -93,6 +93,9 @@ uint8_t matrix_scan(void)
|
||||
#endif
|
||||
if (key == M0110_NULL) {
|
||||
return 0;
|
||||
} else if (key == M0110_ERROR) {
|
||||
// TODO: error recovery or reinit
|
||||
return 0;
|
||||
} else {
|
||||
#ifdef MATRIX_HAS_LOCKING_CAPS
|
||||
if (host_keyboard_leds() & (1<<USB_LED_CAPS_LOCK)) {
|
||||
|
9
rules.mk
9
rules.mk
@ -230,6 +230,8 @@ LDFLAGS += $(EXTMEMOPTS)
|
||||
LDFLAGS += $(patsubst %,-L%,$(EXTRALIBDIRS))
|
||||
LDFLAGS += $(PRINTF_LIB) $(SCANF_LIB) $(MATH_LIB)
|
||||
#LDFLAGS += -T linker_script.x
|
||||
# You can give EXTRALDFLAGS at 'make' command line.
|
||||
LDFLAGS += $(EXTRALDFLAGS)
|
||||
|
||||
|
||||
|
||||
@ -315,9 +317,10 @@ GENDEPFLAGS = -MMD -MP -MF .dep/$(@F).d
|
||||
|
||||
# Combine all necessary flags and optional flags.
|
||||
# Add target processor to flags.
|
||||
ALL_CFLAGS = -mmcu=$(MCU) -I. $(CFLAGS) $(GENDEPFLAGS)
|
||||
ALL_CPPFLAGS = -mmcu=$(MCU) -I. -x c++ $(CPPFLAGS) $(GENDEPFLAGS)
|
||||
ALL_ASFLAGS = -mmcu=$(MCU) -I. -x assembler-with-cpp $(ASFLAGS)
|
||||
# You can give EXTRAFLAGS at 'make' command line.
|
||||
ALL_CFLAGS = -mmcu=$(MCU) -I. $(CFLAGS) $(GENDEPFLAGS) $(EXTRAFLAGS)
|
||||
ALL_CPPFLAGS = -mmcu=$(MCU) -I. -x c++ $(CPPFLAGS) $(GENDEPFLAGS) $(EXTRAFLAGS)
|
||||
ALL_ASFLAGS = -mmcu=$(MCU) -I. -x assembler-with-cpp $(ASFLAGS) $(EXTRAFLAGS)
|
||||
|
||||
|
||||
|
||||
|
Loading…
Reference in New Issue
Block a user